Tips and Tricks on Mastering Common C# Coding Interview Questions
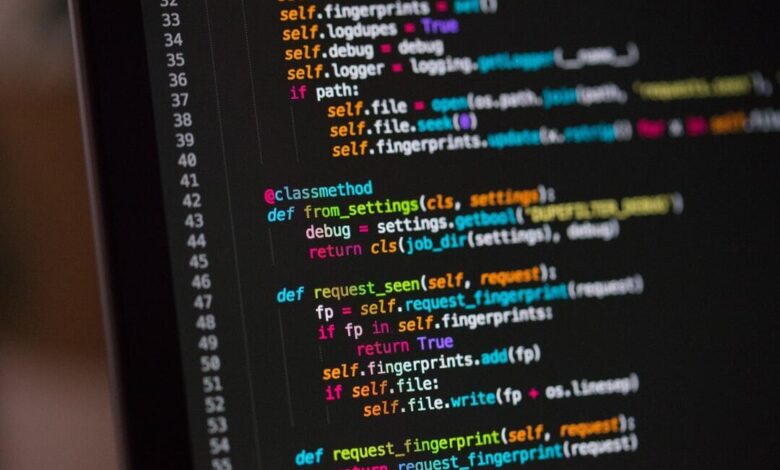
Are you gearing up for a C# coding interview? Whether you’re a seasoned developer or a recent graduate, preparing for technical interviews can be daunting.
But worry not! This guide is here to help you master common C# coding interview questions, so you can walk into your next interview with confidence.
In this blog post, we’ll cover the essential tips and tricks you need to excel in your C# coding interview. You’ll get insights on the basics and get examples on advanced topics to help you stand out. Let’s get started!
The Importance of C# in Modern Development
C# is a powerful, versatile programming language widely used in the following:
- web development
- game development
- enterprise applications
Knowing C# is a valuable skill that can open doors to many job opportunities. But to secure that dream job, you need to master the common C# interview questions and answers.
Understanding the Basics
Before jumping into complex problems, ensure you have a solid grasp of C# fundamentals. Here’s what you need to focus on:
Data Types and Variables
Knowing the various data types in C# is crucial. Understand the differences between value types. These are int, double, and char. Reference types include string, arrays, and objects. Practice declaring and initializing variables to avoid common pitfalls.
Control Structures
Familiarize yourself with control structures like:
- if-else statements
- switch-case
- loops (for, while, do-while)
- error handling using try-catch blocks
These constructs are often used in c++ coding interview questions to test your logic and problem-solving skills.
Object-Oriented Programming (OOP) Concepts
C# is an object-oriented language, so a strong understanding of OOP concepts like classes, objects, inheritance, polymorphism, encapsulation, and abstraction is essential. Be ready to explain these concepts and provide examples during your interview.
Mastering Common C# Interview Questions
Once you have the basics down, it’s time to tackle common embedded c interview questions. Here are some tips and tricks to help you excel:
Arrays and Collections
Arrays and collections are frequently used in coding interviews. Practice creating, manipulating, and iterating through arrays, lists, dictionaries, and other collections. Understand the differences between them and when to use each one.
String Manipulation
String manipulation is a common topic in coding interviews. Be prepared to reverse strings, check for palindromes, and handle various string operations using built-in methods and custom algorithms.
Algorithms and Data Structures
Having a strong grasp of algorithms and data structures is key to success in technical interviews. Focus on the following:
Sorting Algorithms
Understand different sorting algorithms like bubble sort, quicksort, and mergesort. Be able to explain their time complexities, such as O(n^2) for bubble sort and O(n log n) for quicksort and mergesort. Practice implementing these algorithms in C# and understand their best, average, and worst-case scenarios.
Searching Algorithms
Practice implementing linear search and binary search algorithms. Know the differences between them, such as linear search being O(n) and binary search being O(log n), which require a sorted array.
Understand their time complexities and when it is most efficient to use each one. Implement these searches in C# and test them with different datasets.
Data Structures
Familiarize yourself with a variety of data structures like linked lists, stacks, queues, trees, and graphs. Understand their properties, real-world applications, and how to perform operations such as insertion, deletion, and traversal. Practice implementing these structures in C# and work on manipulating them to solve common programming problems efficiently.
Advanced Topics and Techniques
To truly stand out in your interview, you need to demonstrate your knowledge of advanced C# topics and techniques. Here are some areas to focus on:
LINQ (Language-Integrated Query)
LINQ is a powerful feature in C# that allows you to write queries directly within your code, combining the querying capabilities of SQL with the flexibility of C#. Practice using LINQ to perform complex data manipulations and queries on collections, such as filtering, ordering, and grouping data. This will not only simplify your code but also make it more readable and maintainable.
Asynchronous Programming
Understanding asynchronous programming is crucial for modern C# development, especially when dealing with I/O-bound operations like file access or network calls. Practice using async and await keywords to write asynchronous methods and handle tasks efficiently. This helps in keeping the application responsive and improves performance by preventing the blocking of threads.
Design Patterns
Familiarize yourself with common design patterns like Singleton, Factory, Observer, and Strategy. These patterns provide solutions to common software design problems and help in creating more scalable and maintainable code.
Be ready to discuss their use cases, advantages, and disadvantages, and implement them in C#. Understanding when and how to apply these patterns is key to becoming a proficient software developer.
Practical Tips for Interview Day
Preparation is key, but your performance on the day of the interview also matters. Here are some practical tips to help you succeed:
Practice, Practice, Practice
The more you practice, the more comfortable you’ll be during the interview. Use online coding platforms like LeetCode, HackerRank, or CodeSignal to solve a wide variety of practice problems.
Additionally, take mock interviews with friends or use platforms like Pramp to simulate real interview conditions. This will help build your confidence and improve your problem-solving speed.
Think Aloud
During the interview, verbalize your thought process as you work through problems. Clearly explain your reasoning, the steps you are taking, and any assumptions you are making. This helps the interviewer understand your approach and problem-solving skills, and it also allows them to provide guidance if you are heading in the wrong direction.
Stay Calm and Focused
Interviews can be stressful, but staying calm and focused is essential for performing well. Take a deep breath before you start, read the problem carefully to fully understand it, and break it down into smaller, manageable steps.
If you get stuck, don’t panic; take a moment to reassess the problem and think of alternative approaches. Remember, how you handle challenges during the interview can be just as important as finding the correct solution.
Master The Common C# Coding Interview Questions Today
Mastering common C# coding interview questions is no small feat. But with the right preparation and practice, you can ace your next interview.
Focus on understanding the basics, practicing common questions, and tackling advanced topics. Remember to stay calm, think aloud, and showcase your problem-solving skills.
Ready to take your C# skills to the next level? Start practicing today and prepare to impress your interviewers. Good luck!
Looking for more fascinating reads? Check out our blog for a plethora of articles on a multitude of topics. Happy reading!